A snapshot test utility to simplify your tests
Snapshooter is a flexible .Net testing tool to simplify the validation of your test results in your unit / integration tests. It creates simply a snapshot of your test result and stores it alongside of your test. When the test is executed again, the snapshooter will compare the actual test result with the stored snapshot. If both are the same, the test will pass.
Snapshooter is based on the idea of Jest Snapshot Testing
// arrange
var serviceClient = new ServiceClient();
// act
TestPerson person = serviceClient.CreatePerson(...);
// assert
Assert.IsEqual(person.Firstname, "David");
Assert.IsEqual(person.Lastname, "Smith");
Assert.IsEqual(person.Age, 30);
Assert.IsEqual(person.Size, 182.5214);
Assert.IsEqual(person.CreationDate, DateTime.Parse(...));
Assert.IsEqual(person.DateOfBirth, DateTime.Parse(...));
Reduce your asserts in your test
Reduce your asserts in your test
Instead of writing multiple asserts within your test assert section, just reduce it to one assert.
Sometimes we receive a test result object with several properties, and all of them have to be checked... Now instead of writing for each property an assert, just validate all properties at once with a snapshot.
// arrange
var serviceClient = new ServiceClient();
// act
TestPerson person = serviceClient.CreatePerson(...);
// assert
Snapshot.Match(person);
Get started in seconds
Get started in seconds
With Snapshooter you need just two commands to get a snapshot test validation running in your test project.
Install the snapshooter nuget package within your test project:
dotnet add package Snapshooter.Xunit
Assert the result in your test with the Snapshot.Match command:
[Fact]
public void CreatePerson_VerifyPersonBySnapshot_SuccessfulVerified()
{
// arrange
var serviceClient = new ServiceClient();
// act
TestPerson person = serviceClient.CreatePerson(
Guid.Parse("2292F21C-8501-4771-A070-C79C7C7EF451"), "David", "Mustermann");
// assert
Snapshot.Match(person);
}
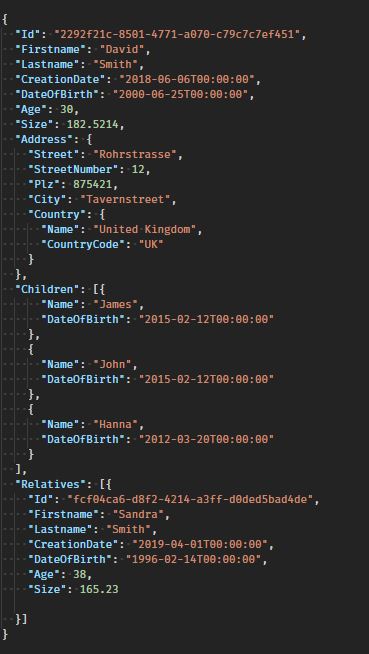